User Accounts
Overview
User accounts can be created for internal and external purposes, whether it's an employee or a customer. They can be created within the Main Account and within Sub-Accounts. The following diagram illustrates this concept:
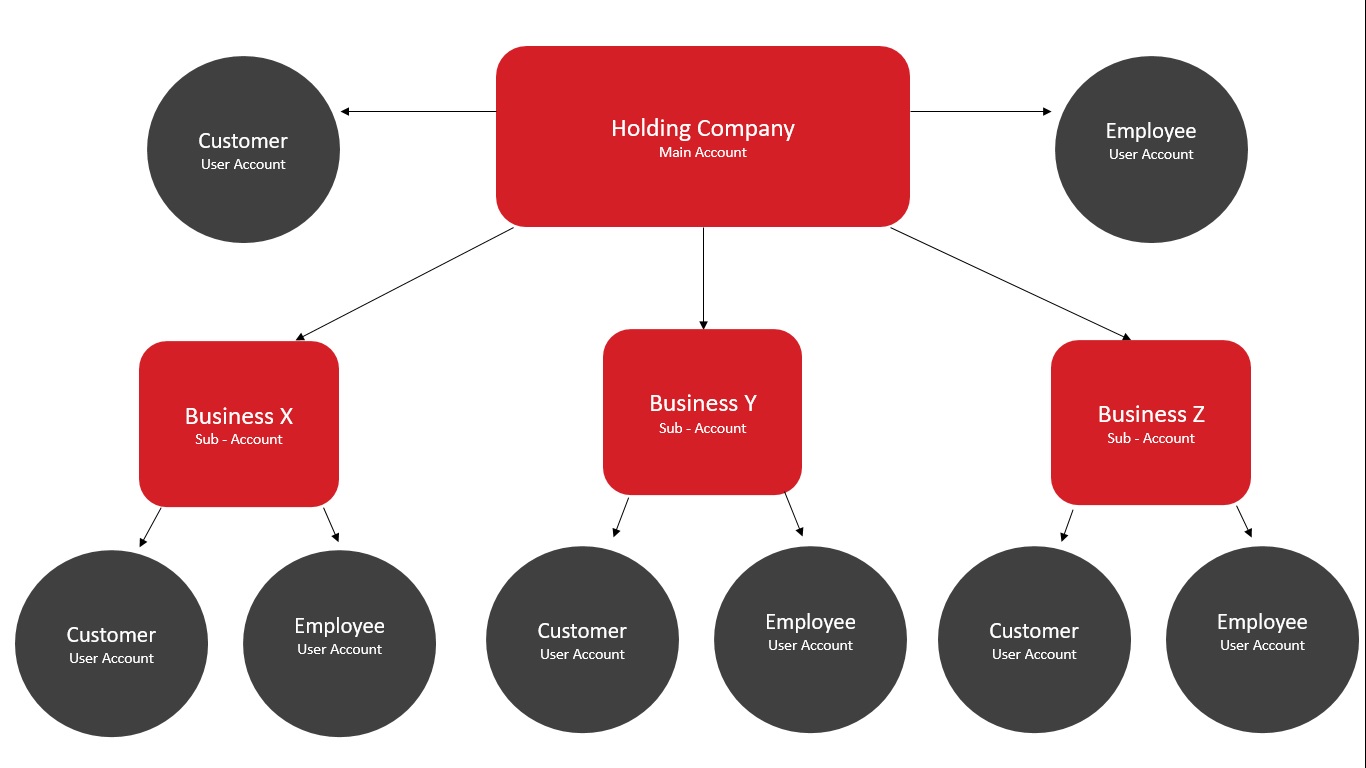
Create a User Account
To create a User account:
- Call
POST - Create Users
. - For
Password
enter a cleartext password.
REQUEST
For a typical request the following information must be completed before being submitted.
curl --location 'https://caxapi-test.azurewebsites.net/api/registration//third-party/onboarding' \
--header 'Content-Type: application/json' \
--header 'Authorization: bearer <accesstoken>' \
--header 'IvKey: <IvKey>' \
--data-raw '{
"Email": "[email protected]",
"FirstName": "John",
"LastName": "Caxton",
"Gender":"Male",
"Title": "Mr",
"Password": "Admin@123",
"MemorableWord":"aaa",
"DateofBirth": "1990-05-20",
"HouseNameNumber": "Downway",
"Address1":"2 Lehman Street",
"City":"London",
"Postcode": "E18FA",
"Country":"GB",
"Product":"MoneyTransfer",
"PrimaryContactNo":"+447686786786",
"MobileNumber":"+447437570777",
"DeviceId":"<DeviceId>",
"Device":"<Device>",
"OperatingSystem":"iOS 12.2",
"AppVersion": "8.1.2"
}
'
RESPONSE
A successful response creates an ApplicantId
:
{
"Content": {
"Model": {
"ApplicantId": 12345,
"Userid": "<userid>",
"ApiToken": "<apitoken>",
"ApiStatusCode": 314,
"ApiStatus": "Success",
"ApiStatusDescription": "The application has been successful."
},
"ExpectedResponses": [
"Success"
]
User Login
To log in:
- Call
POST - User Login
. - For
UserName
enter the email address used to register the account. Password
must be hashed using md5 then converted to a hex before being packaged as base64. The following snippets are available:
using System;
using System.Security.Cryptography;
using System.Text;
public class Program
{
public static void Main()
{
Console.WriteLine(PasswordHash("Admin@123"));
}
public static string PasswordHash(string password)
{
using (MD5 md5 = MD5.Create())
{
byte[] inputBytes = Encoding.ASCII.GetBytes(password);
byte[] hashBytes = md5.ComputeHash(inputBytes);
StringBuilder sb = new StringBuilder();
for (int i = 0; i < hashBytes.Length; i++)
{
sb.Append(hashBytes[i].ToString("X2"));
}
return sb.ToString();
}
}
}
#include <stdio.h>
#include <string.h>
#include <openssl/md5.h>
void password_hash(char *password, char *output) {
unsigned char digest[16];
MD5_CTX ctx;
MD5_Init(&ctx);
MD5_Update(&ctx, password, strlen(password));
MD5_Final(digest, &ctx);
for(int i = 0; i < 16; ++i)
sprintf(&output[i*2], "%02x", (unsigned int)digest[i]);
}
int main() {
char output[33];
password_hash("Admin@123", output);
printf("%s\n", output);
return 0;
}
#include <iostream>
#include <openssl/md5.h>
std::string password_hash(std::string password) {
unsigned char digest[MD5_DIGEST_LENGTH];
MD5((unsigned char*)password.c_str(), password.size(), (unsigned char*)&digest);
char mdString[33];
for(int i = 0; i < 16; i++)
sprintf(&mdString[i*2], "%02x", (unsigned int)digest[i]);
return std::string(mdString);
}
int main() {
std::cout << password_hash("Admin@123") << std::endl;
return 0;
}
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Base64;
public class Main {
public static void main(String[] args) throws NoSuchAlgorithmException {
System.out.println(passwordHash("Admin@123"));
}
public static String passwordHash(String password) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance("MD5");
md.update(password.getBytes());
byte[] digest = md.digest();
return Base64.getEncoder().encodeToString(digest);
}
}
// Hex Encryption Algorithm
function hex2a(hexx) {
var hex = hexx.toString(); //force conversion
var str = '';
for (var i = 0; i < hex.length; i += 2) {
str += String.fromCharCode(parseInt(hex.substr(i, 2), 16));
}
return str;
}
// Password Hashing Algorithm
function passwordHash(password) {
var hashed = hex2a(md5(password));
return btoa(hashed);
}
console.log(passwordHash('Admin@123'));
<?php
// Hex Encryption Algorithm
function hex2a($hexx) {
$hex = strval($hexx); //force conversion
$str = '';
for ($i = 0; $i < strlen($hex); $i += 2) {
$str .= chr(hexdec(substr($hex, $i, 2)));
}
return $str;
}
// Password Hashing Alorithm
function passwordHash($password) {
$hashed = hex2a(md5($password));
return base64_encode($hashed);
}
echo passwordHash('Admin@123');
extern crate crypto;
extern crate base64;
use crypto::digest::Digest;
use crypto::md5::Md5;
fn password_hash(password: &str) -> String {
let mut hasher = Md5::new();
hasher.input_str(password);
let hashed = hasher.result_str();
return base64::encode(&hashed);
}
fn main() {
println!("{}", password_hash("Admin@123"));
}
- For
Authorization
, typebearer
, add a space, and enter the access token .
REQUEST
curl --location 'https://caxapi-test.azurewebsites.net/api/users/auth/login-step-one' \
--header 'Content-Type: application/json' \
--header 'Authorization: bearer <accesstoken>' \
--data-raw '{
"username": "<username>",
"Password": "<Password>",
"DeviceId": "<DeviceId>",
"Device": "<Device>"
}
'
RESPONSE
{
"Content": {
"Model": {
"LoginToken": "<LoginToken>",
"TokenStartDate": "2024-02-09T00:00:00",
"TokenEndDate": "2024-02-10T00:00:00",
"Details": {
"Id": "<Id>",
"Title": "Ms",
"FirstName": "User",
"LastName": "Test",
"Gender": "Other",
"Address1": "The House",
"Address2": null,
"City": "London",
"County": null,
"Postcode": "N76FJ",
"Country": "GB",
"ContactEmail": "[email protected]",
"PhoneNumber": "75565645644",
"MobileNumber": "+447666778899",
"DefaultCurrency": "EUR",
"ReferralCode": null,
"Email": "[email protected]",
"MobilePhoneValidationStatus": 260
},
"Claims": {
"IsCorporateDomesticAccount": false,
"IsCorporateDomesticRestrictATMAccount": false,
"IsCorporateAccount": true,
"CorporateEntityId": 0000,
"CorporateEntityName": "renameofmyco",
"CorporateIdentityNumber": "1234567890",
"ForcePasswordReset": false,
"CanAccessCurrencyCards": true,
"CanAccessMoneyTransfer": true,
"OptedInForCommunications": false,
"IPDataCaptureRequired": false,
"ApiStatusCode": 100,
"ApiStatus": "IsValid",
"ApiStatusDescription": "Valid Operation"
},
"PushNotificationStatus": 0,
"ApiStatusCode": 210,
"ApiStatus": "LoginSuccess",
"ApiStatusDescription": "Login successful."
},
"ExpectedResponses": [
"LoginSuccess",
"LoginTokenGenerated",
"LoginSuccessRedAccountSignup",
"LoginSuccessIPAccountSignup",
"LoginSuccessEmailNotConfirmed"
]
},
"AuthorisedClientModel": {
"ClientId": "<clientid>",
"ClientRef": "<clientref>",
"UserId": "<userid>",
"TokenStartDate": "2024-01-09T09:39:00.5756167",
"TokenEndDate": "2024-02-11T09:50:44.0200074",
"ApiStatusCode": 100,
"ApiStatus": "IsValid",
"ApiStatusDescription": "Valid Operation"
},
"AuthorisedUserModel": {
"UserId": "<userid>",
"AppVersion": "20240206.2",
"TokenStartDate": "2024-02-09T00:00:00",
"TokenEndDate": "2024-02-10T00:00:00",
"LoginTimestamp": "2024-02-09T09:50:43.7543498",
"ApiLoginType": 1,
"ApiStatusCode": 100,
"ApiStatus": "IsValid",
"ApiStatusDescription": "Valid Operation"
}
}
Get User Details
When information about a particular User must be retrieved.
- Call
Get - User Details
.
REQUEST
curl --location 'https://caxapi-test.azurewebsites.net/api/users/user/account/details' \
--header 'Authorization: bearer <accesstoken>' \
--header 'UserApiToken: ' \
--header 'Content-Type: application/json' \
--data ''
A typical response is highlighted below:
RESPONSE
{
"MainAccountHolderName": "[email protected]",
"Content": {
"Model": {
"Id": "<Id>",
"Title": "Ms",
"FirstName": "User",
"LastName": "Test",
"Gender": "Other",
"Address1": "The House",
"Address2": null,
"City": "London",
"County": null,
"Postcode": "N76FJ",
"Country": "GB",
"ContactEmail": "[email protected]",
"Email": "[email protected]",
"PhoneNumber": "75565645644",
"MobileNumber": "+447666778899",
"ReferralCode": "",
"PaymentReference": "1234567890",
"MobilePhoneValidationStatus": 260,
"ApiStatusCode": 100,
"ApiStatus": "IsValid",
"ApiStatusDescription": "Valid Operation"
},
"ExpectedResponses": [
"IsValid"
]
},
"AuthorisedClientModel": {
"ClientId": "<clientid>",
"ClientRef": "<clientref>",
"UserId": "<userid>",
"TokenStartDate": "2024-01-09T09:39:00.5756167",
"TokenEndDate": "2024-02-11T09:50:44.0200074",
"ApiStatusCode": 100,
"ApiStatus": "IsValid",
"ApiStatusDescription": "Valid Operation"
},
"AuthorisedUserModel": {
"UserId": "<userid>",
"AppVersion": null,
"TokenStartDate": "2024-02-09T00:00:00",
"TokenEndDate": "2024-02-10T00:00:00",
"LoginTimestamp": "2024-02-09T09:50:49.5507649",
"ApiLoginType": 0,
"ApiStatusCode": 100,
"ApiStatus": "IsValid",
"ApiStatusDescription": "Valid Operation"
}
}
Updated 6 days ago